❗ This is not a tutorial ❗
Here’s a basic implementation:
$ mkdir app/components
$ touch app/components.Header.tsx
// app/components/Header.tsx
import { Link } from "@remix-run/react";
export default function Header() {
return (
<header>
<h1>
<Link to="/">Hello Zalgorithm</Link>
</h1>
</header>
);
}
Add the header to root.tsx
. …I’m skipping a few things here. The idea is that all of the site’s routes are rendered through the <Outlet />
component that’s now below the <Header />
component in app/root.tsx
:
// app/root.tsx
import type { LinksFunction } from "@remix-run/node";
import {
Links,
LiveReload,
Meta,
Outlet,
Scripts,
ScrollRestoration,
} from "@remix-run/react";
import Header from "~/components/Header";
import styles from "./tailwind.css";
export const links: LinksFunction = () => [{ rel: "stylesheet", href: styles }];
export default function App() {
return (
<html lang="en">
<head>
<meta charSet="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<Meta />
<Links />
</head>
<body>
<Header />
<Outlet />
<ScrollRestoration />
<Scripts />
<LiveReload />
</body>
</html>
);
}
It needs some styling:
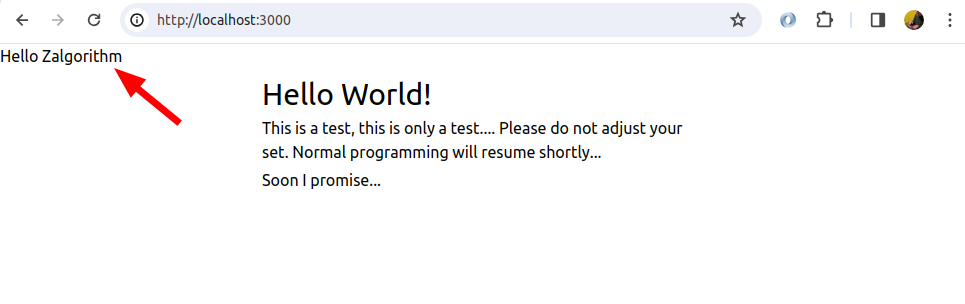
The easiest way to see what’s going on with an HTML element is to add a background color.
Heading to the Tailwind docs:
I’ll give this a try:
// app/components/Header.tsx
import { Link } from "@remix-run/react";
export default function Header() {
return (
<header className="bg-slate-500 text-slate-50">
<h1>
<Link to="/">Hello Zalgorithm</Link>
</h1>
</header>
);
}
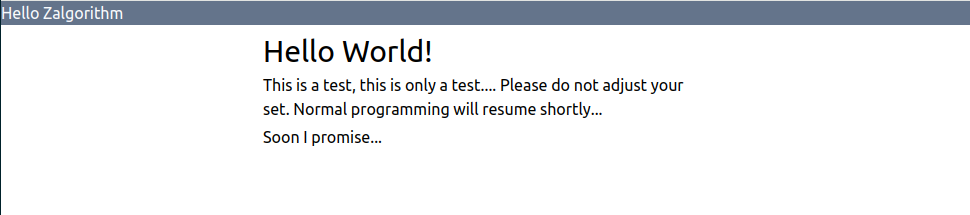
Getting somewhere! The text needs to be bigger and could use some padding. I think I’ll keep it on the left of the page. There needs to be some room to add Log In/Log Out links, and maybe a drop down menu on the right.
Back to the Tailwind docs:
Maybe:
<header className="bg-slate-500 text-slate-50 text-xl px-3 py-1">
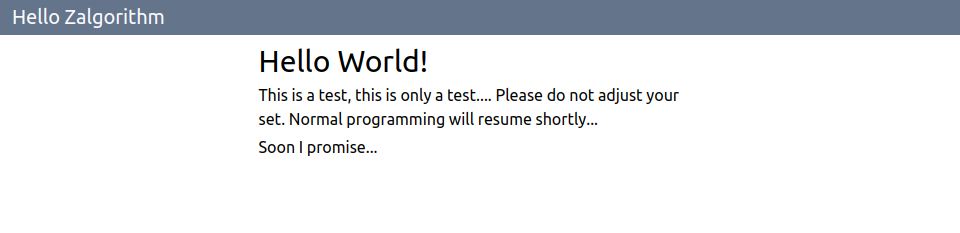
Nicely understated, but I’ve got to be able to fit some buttons in there. How about className="bg-slate-500 text-slate-50 text-xl p-3"
?
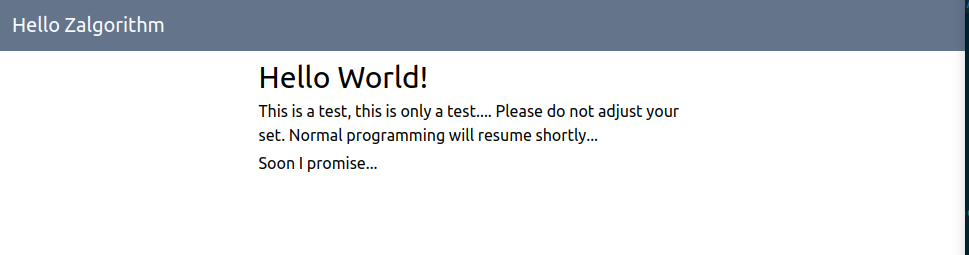
Good enough for now. I think that for wider screens, I’m going to add a sidebar to the left of the page. That should balance things out a bit.
Things won’t stay this way, but I’ve added a “Blogs” link to the header:
// app/components/Header.tsx
import { Link } from "@remix-run/react";
export default function Header() {
return (
<header className="bg-slate-500 text-slate-50 text-xl p-3 flex justify-between items-center">
<h1>
<Link to="/">Hello Zalgorithm</Link>
</h1>
<div>
<Link to="/blogs">Blogs</Link>
</div>
</header>
);
}
I’m using a couple of Tailwind classes to align the items horizontally and vertically (vertical alignment will become an issue when buttons are added to the header.)
flex
(css:display: flex
). This applies the Flexbox layout to the header. The header’s direct children (h1
anddiv
) are now flex items.justify-between (
css:justify-content: space-between
). Spaces the flex items within the container with the maximum amount of space between them.items-center
(css:align-items: center
). Aligns the flex items vertically.
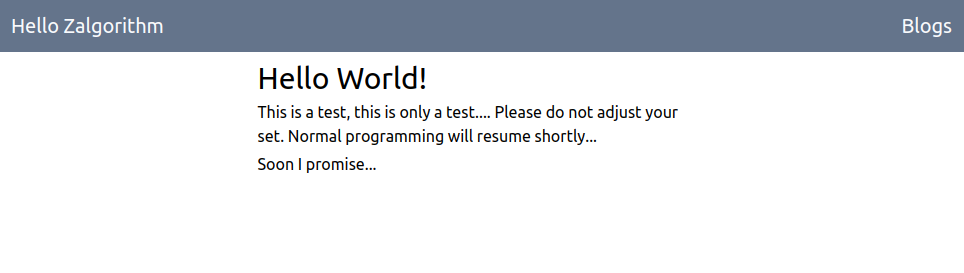
Might as well push this to the production site:
$ git add .
$ git commit -m "Add a header component"
$ git push live main
$ ssh <redacted path to the live server>
# on the production site
$ cd /var/www/hello_zalgorithm_com
# I haven't added any node modules, but it doesn't hurt to run:
$ npm install
up to date, audited 837 packages in 2s
245 packages are looking for funding
run `npm fund` for details
found 0 vulnerabilities
# then
$ npm run build
# I know the id of the pm2 instance is 0, so I can go ahead and run:
$ pm2 reload 0
Success 🙂 https://hello.zalgorithm.com/
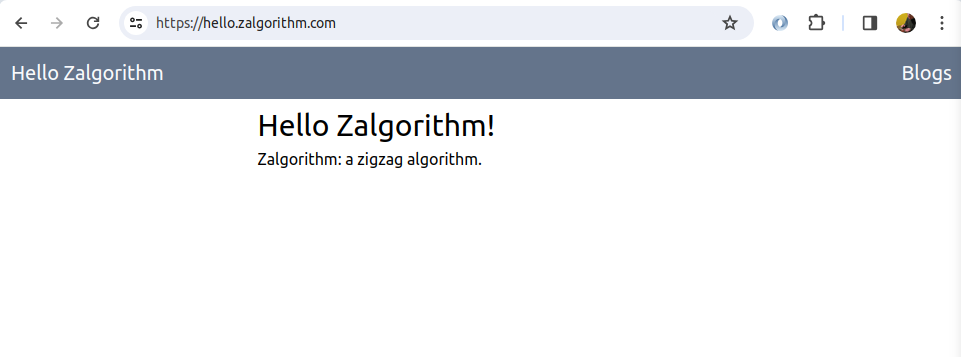
Todo:
- I’d like to find a better way of dealing with code snippets in posts. Holding off on that because this whole blog is moving over to Remix – I’ll still use WordPress for its database though.
- I’m asking questions in my posts that I don’t have the answers to. I’d like to find a way to mark a section of text as a question. Maybe create a
question
custom post type? That could give me a list of questions atzalgorithm.com/questions
. - I need to use more headings in my posts. Anchor links would be a good idea too.